I am thinking about a converter from bitmaps fonts to a lisp-format describing the needed Pixelgrid
Example letter bitmap 7 x 15 (zoomed):
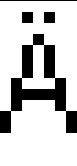
(//%3C/s%3E%3CURL%20url=%22http://hpwsoft.de/anmeldung/html1/newLISP/CharGrid.png%22%3Ehttp://hpwsoft.de/anmeldung/html1/newLISP/CharGrid.png%3C/URL%3E%3Ce%3E)
I scan the bitmap vertical and horzontal and get 2 vector lists.
Bitmap: 7 x 15 Pixel
Vertical scan:
; X1 Y1 X2 Y2 Length
(list 0 10 0 11) ;2
(list 1 7 1 9) ;3
(list 2 1 2 1) ;1
(list 2 4 2 6) ;3
(list 2 8 2 8) ;1
(list 3 3 3 3) ;1
(list 3 8 3 8) ;1
(list 4 1 4 1) ;1
(list 4 4 4 6) ;3
(list 4 8 4 8) ;1
(list 5 7 5 9) ;3
(list 6 10 6 11) ;2
Horizontal scan:
; X1 Y1 X2 Y2 Length
(list 2 1 2 1) ;1
(list 4 1 4 1) ;1
(list 3 3 3 3) ;1
(list 2 4 2 4) ;1
(list 4 4 4 4) ;1
(list 2 5 2 5) ;1
(list 4 5 4 5) ;1
(list 2 6 2 6) ;1
(list 4 6 4 6) ;1
(list 1 7 1 7) ;1
(list 5 7 5 7) ;1
(list 1 8 5 8) ;5
(list 1 9 1 9) ;1
(list 5 9 5 9) ;1
(list 0 10 0 10) ;1
(list 6 10 6 10) ;1
(list 0 11 0 11) ;1
(list 6 11 6 11) ;1
So I want to draw the pixels with the vectors with the largest length and want to avoid double vectors.
Wanted Result:
(list 0 10 0 11) ;2
(list 1 7 1 9) ;3
(list 2 1 2 1) ;1
(list 2 4 2 6) ;3
(list 3 3 3 3) ;1
(list 4 1 4 1) ;1
(list 4 4 4 6) ;3
(list 5 7 5 9) ;3
(list 6 10 6 11) ;2
(list 1 8 5 8) ;5
Any lispish ideas?
After thinking about it my first idea is:
I build a list with all vectors and sort it after the vector lenght.
Then I start with the longest vectors and build a second list
with the setted single pixels. Every new vector is checked if it will set any new pixel.
If not it will be dropped from the result-vector list.
I will try this out.
How about a list of coordinate pairs:
'((0 10) (0 11))
I can see this being useful sometimes...
My current implementation of the optimising part:
(The sublists in resultlist have now an additional value for the vector direction)
(setq pixellist '()
resultlist2 '()
)
(dolist (vlst resultlist)
(setq vectordir(nth 1 vlst)
vectorstart(nth 0(nth 2 vlst))
vectorend(nth 1(nth 2 vlst))
)
(if (= vectordir 0) ;X
(begin
;Check if vector is needed
(setq VectorIsNeeded nil)
(for (x(first vectorstart)(first vectorend)1)
(if (not(member (list x (last vectorstart)) pixellist))
(setq VectorIsNeeded true)
)
)
(if VectorIsNeeded
(begin
(setq resultlist2 (append resultlist2 (list vlst)))
;Add all pixel to pixellist
(for (x(first vectorstart)(first vectorend)1)
(if (not(member (list x (last vectorstart)) pixellist))
(setq pixellist (append pixellist (list (list x (last vectorstart)))))
)
)
)
)
)
)
(if (= vectordir 1) ;Y
(begin
;Check if vector is needed
(setq VectorIsNeeded nil)
(for (y(last vectorstart)(last vectorend)1)
(if (not(member (list (first vectorstart) y) pixellist))
(setq VectorIsNeeded true)
)
)
(if VectorIsNeeded
(begin
(setq resultlist2 (append resultlist2 (list vlst)))
;Add all pixel to pixellist
(for (y(last vectorstart)(last vectorend)1)
(if (not(member (list (first vectorstart) y) pixellist))
(setq pixellist (append pixellist (list (list (first vectorstart) y))))
)
)
)
)
)
)
)